The Most Common Programming Interview Questions You’ll Get Asked
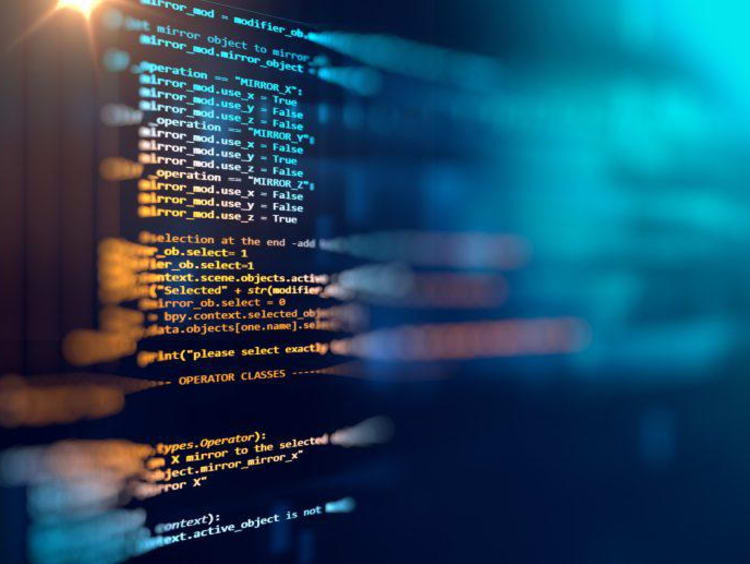
Computer programmers can make a great impression at the technical interview by being well-prepared for the most common questions. Typically, coders spend weeks studying before each interview. This might seem excessive but remember that the more time you spend preparing for the questions, the more confident you’ll feel in front of the whiteboard.
Questions About Arrays
Interviewers love to ask questions about arrays. In a typical technical interview, you’ll likely get asked multiple questions about them. The problem with them is that, once an array is created, it is impossible to change its size. If you need to make an array shorter or longer, you’ll have to create a brand new array and transfer the elements. To answer array-based questions proficiently, you should brush up on array data structure and programming constructors, like fundamental operators. Consider these common array-based questions and directives:
- Using Java, reverse an array in place.
- If an array has multiple duplicates, how can you find duplicate numbers?
- Using Java, remove duplicates from a particular array.
- Identify the smallest and largest numbers on an unsorted integer array.
Questions About Linked Lists
Like an array, a linked list stores elements in a linear way. Unlike the array, a linked list does not rely on contiguous storage locations for the elements. Since linked lists are just lists of nodes, you can add or take away elements instead of creating an entirely new linked list. Linked lists are recursive data structures so brush up on the basics of recursion before your technical interview. Consider these common linked list questions and directives:
- Reverse a linked list.
- Without recursion, reverse a singly linked list.
- How can you eliminate duplicate nodes in an unsorted linked list?
- How can you convert a binary tree to a doubly linked list?
- How can you swap every two nodes?
Questions About String Coding
If you have a solid knowledge of the array, string-based questions should be easier for you. Strings are a character array. It can be helpful if you remind yourself of the structure of strings, regardless of which programming language you’re using for the solutions. And always remember that strings are immutable. Some of the most common string-based questions and directives include:
- Find all the permutations of a string.
- How can you use recursion to reverse a given string?
- Are two given strings a rotation of each other?
- Is a given string a palindrome?
- How can you determine if two strings are anagrams of each other?
- How could you print duplicate characters from a given string?
- How can you tell if there are only digits on a given string?
As you can see, there are lots of questions you should prepare for when you’re getting ready for your technical interview. Set aside more time to study than you think you’ll need.
You can prepare for a seamless transition from academia into the workforce with help from the Career IMPACT Center and the Office of Internships. Grand Canyon University is committed to helping our students achieve their career aspirations. If you’re a future student who wants to learn more about our Bachelor of Science in Computer Programming degree, you can click on the link to Request More Information.
The views and opinions expressed in this article are those of the author’s and do not necessarily reflect the official policy or position of Grand Canyon University. Any sources cited were accurate as of the publish date.